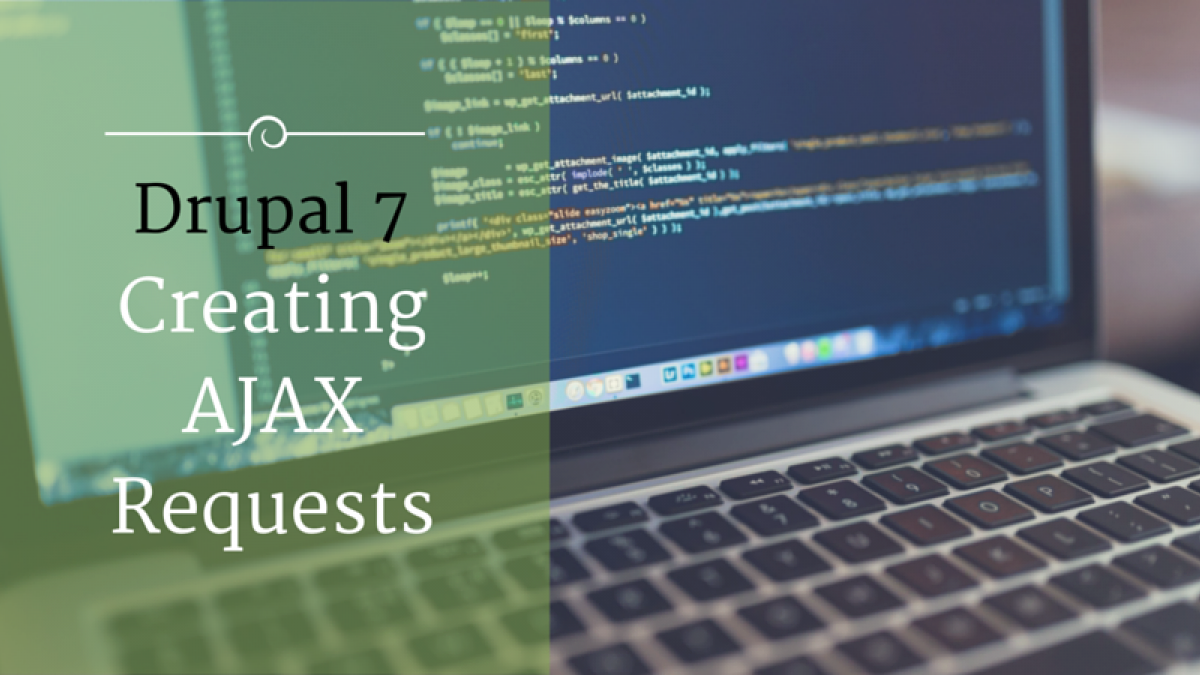
Creating AJAX Requests with Drupal 7
AJAX is a method of sending/receiving data on a web page. It can be used to update parts of the web page like little pieces of content, blocks and whatsoever, without the need to reload the page completely. It could be also used to submit forms, transfer or exchange data in real time.
In this blog post, I'm going to show you how to use Ajax to update some of the page content. This is going to require some Javascript coding, an empty custom module, and some html.
Let's define the scope of this example. We will create a page that has a button, once the button is created we will request a number of List Items to be displayed and placed on the page right below it.
Let's start with some simple HTML We only have a button that triggers the AJAX call, and a div wrapper where we are going to place the AJAX result. This HTML markup can go on a node or Drupal basic page:
<button class="btn btn-md btn-success">Retrieve list items</button> <div id="ajax-result"></div>
Now, let's setup the custom module which simply has a menu callback that returns a number of list items, based on the AJAX request.
<?php function custom_init() { drupal_add_js(array('custtom' => array('ajaxUrl' => url('custom/ajax'))), 'setting'); drupal_add_js(drupal_get_path('module', 'custom') . '/custom.js'); } function custom_menu() { $items['custom/ajax'] = array( 'type' => MENU_CALLBACK, 'page callback' => 'custom_ajax', 'access arguments' => array('access content'), ); return $items; } function custom_ajax() { $numberOfItems = (isset($_GET['numberOfItems']) ? $_GET['numberOfItems'] : 10); $output = '<ul>'; for($i = 0; $i < $numberOfItems; $i++) { $output .= "<li> List item number {$i} </li>"; } $output .= '</ul>'; print $output; exit; }
Next, we define the AJAX call in Javascript. We will place the Javascript file inside the module, and name it custom.js, and the code will be wrapped within a Drupal Javascript Behavior that would look like this:
(function ($) { Drupal.behaviors.ajaxExample = { attach: function (context, settings) { // CSS Selector for the button which will trigger the AJAX call $('.btn', context).click(function () { $.ajax({ url: Drupal.settings.custom.ajaxUrl, // This is the AjAX URL set by the custom Module method: "GET", data: { numberOfItems : 10 }, // Set the number of Li items requested dataType: "html", // Type of the content we're expecting in the response success: function(data) { $('#ajax-result').html(data); // Place AJAX content inside the ajax wrapper div } }); }); } }; }(jQuery));
This is one of the simplest AJAX implementations for returning straight HTML content. The same method can be used for returning raw data and variables like arrays and objects, and this would require using
drupal_json_output($var = NULL) instead of print in the very last couple of lines in the menu callback.
Also in the Javascript, the datatype would be "json" instead of "html". This way we will receive the AJAX response as a JSON object that we can traverse and operate on.