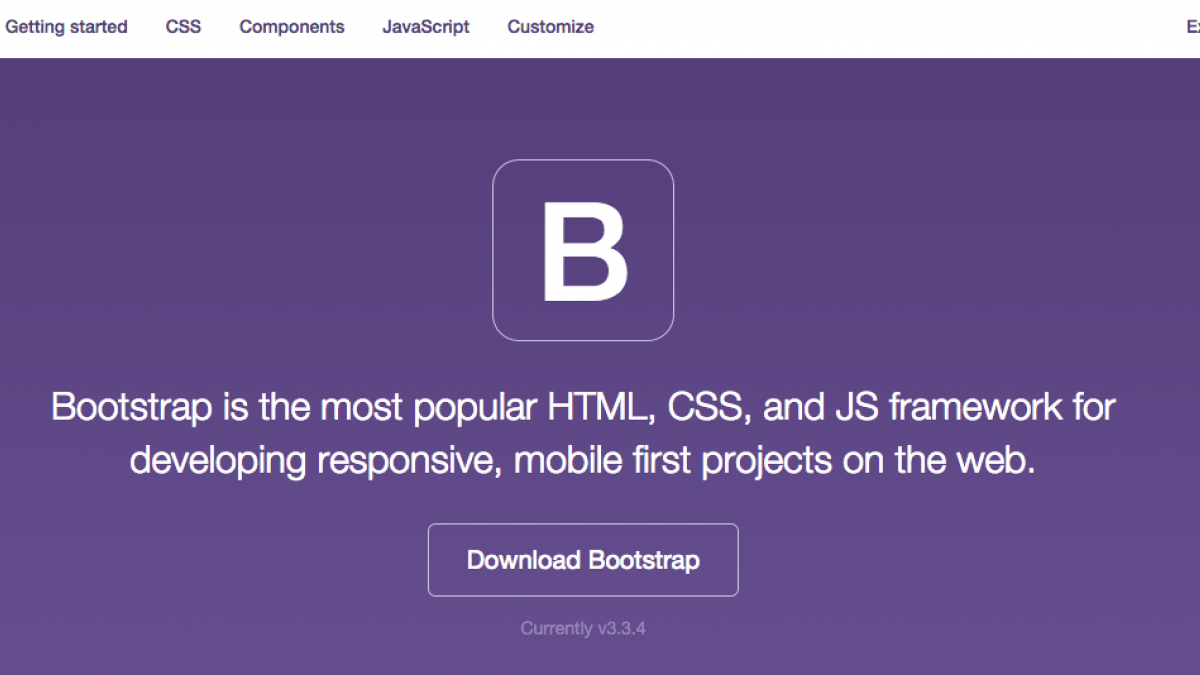
How to use Drupal Bootstrap with Webforms
If you are sub-theming Drupal Bootstrap, you are probably spoiled by all of the awesome functionality that comes with the Bootstrap framework and the Drupal Bootstrap theme. One place where you can’t easily throw a row and col class around your divs through the admin UI is if you are creating a Webform.
I came up with a quick solution to this that, with a little setup, allows the user to leverage Bootstrap through the Webform UI.
What we’re going to accomplish:
Let’s say we’ve built a webform like this:
However, we want the First name, Last name, Email, and Company to be in a two column layout, and Fields 1-3 to be in a three column layout below the body. Using the webform-form.tpl.php file, we will make the form look like this:
How we’re going to do it:
If you check out the webform directory, you’ll see the default webform-form.tpl.php in the templates directory (at something like sites/all/modules/contrib/webform/templates/webform-form.tpl.php). Copy and paste this file into your theme’s directory at [my-subtheme]/templates/webform-form.tpl.php. As the tpl.php says:
<?php // Print out the main part of the form. // Feel free to break this up and move the pieces within the array. print drupal_render($form['submitted']);
What we’ll do is decide upon some Field Keys that will serve as the columns that will contain the fields. Then if we place fields within fieldsets given those Field Keys, they will be displayed within the column. This may not be clear until you look at the code and how it will be used with Webform. In our webform-form.tpl.php, if we use the following code:
<div class="row"> <div class="col-md-6"> <?php print drupal_render($form['submitted']['field_top_left']);?> </div> <div class="col-md-6"> <?php print drupal_render($form['submitted']['field_top_right']); ?> </div> </div>
And then in the webform, create two new fieldsets, Field Top Left and Field Top Right. Make sure to set the Field Key to the field name you used in the webform-form.tpl.php
Nest the First name and Last name fields in Field Top Left fieldset and the Email and Company fields in the Field Top Right fieldset.
I’ve left the fieldset names to display so it’s more clear what’s going on here. Save and view your webform and bam your fields are now in 2 columns.
So the webform-form.tpl.php is picking up the form's Field Keys from the Webform UI that match what is in the template file, and then wrapping them with the necessary Bootstrap markup. We can follow this same pattern for the three column layout. For example in our webform-form.tpl.php, we can use this code:
<div class="row"> <div class="col-md-4"> <?php print drupal_render($form['submitted']['field_lower_third_1']);?> </div> <div class="col-md-4"> <?php print drupal_render($form['submitted']['field_lower_third_2']); ?> </div> <div class="col-md-4"> <?php print drupal_render($form['submitted']['field_lower_third_3']); ?> </div> </div>
Following the pattern above, we can create 3 fieldsets with Field Keys of field_lower_third_1, field_lower_third_2, and field_lower_third_3, and then nest Fields 1-3 in these fieldsets. Alternatively, if you aren't going to add more fields to these fieldsets, you can just give the fields 1-3 the field keys field_lower_third_1 through field_lower_third_3.
If you’re following along, you may notice that any fields you haven’t placed in these fieldsets or given these pre-defined Field Keys are getting pushed to the bottom of the webform, regardless of how they are arranged in the Webform UI.
This is because anything not explicitly declared in the tpl.php is being printed at the bottom in the section that reads:
<?php // Always print out the entire $form. This renders the remaining pieces of the // form that haven't yet been rendered above. print drupal_render_children($form); ?>
Right now this webform-form.tpl.php will also be applied to ALL webforms on the site. There are two ways you can go with this - if you just want to use it for just one webform, rename it webform-form-[nid].tpl.php. However, I think it’s nice for it to be available for all webforms since it’s a handy way to build forms using the Bootstrap layout through the Webform UI.
If you’re going to use it for all forms, you will probably want to wrap your columns in some logic so that the markup is only printed out if these Field Keys are being used. Otherwise you’ll get a lot of empty divs. So we’ll do that for the 2 column:
<?php if((array_key_exists('field_top_left', $form['submitted'])) || (array_key_exists('field_top_right', $form['submitted']))): ?> <div class="row"> <div class="col-md-6"> <?php print drupal_render($form['submitted']['field_top_left']);?> </div> <div class="col-md-6"> <?php print drupal_render($form['submitted']['field_top_right']); ?> </div> </div> <?php endif; ?>
and you can see how then you would do the same for the 3 column layout.
Now we just want to add the Field Key that will contain our Text field at the top of the page
<?php if(array_key_exists('field_top', $form['submitted'])): ?> <div class="row fullwidth"> <div class="col-xs-12"> <?php print drupal_render($form['submitted']['field_top']);?> </div> </div> <?php endif; ?>
and the Field Key that will contain lower body field
<?php if(array_key_exists('field_middle', $form['submitted'])): ?> <div class="row fullwidth"> <div class="col-xs-12"> <?php print drupal_render($form['submitted']['field_middle']);?> </div> </div> <?php endif; ?>
Since we only have 1 field (Body or Texfield) in our full width Field Key elements, we don’t need to use Fieldsets, but can instead just give the Body and Textfields the Field Keys that match with our webform-form.tpl.php.
Check out the webform now, and you’ll see it’s all pretty and Bootstrap-y. Nice.
Have any questions or a different solution? Leave all comments below!